-
Автор темы
- #1
Обратите внимание, пользователь заблокирован на форуме. Не рекомендуется проводить сделки.
Спасибо коню и еексоми за код :P
Запись(создание) модельки и матрицы:
Отрисовка:
Недостающие вещи для многих:
Куда все это записывается:
Результат:
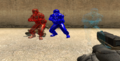
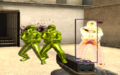
Запись(создание) модельки и матрицы:
Код:
memcpy(info->bones, target->get_bone_cache().base(), sizeof(matrix) * target->get_bone_cache().count());
auto renderable = target->GetClientRenderable();
if (!renderable)
return;
auto model = target->GetModel();
if (!model)
return;
auto hdr = interfaces->model_info->get_studio_model(model);
if (hdr) {
static int m_nSkin = utils->find_in_data_map(target->get_pred_desc_map(), "m_nSkin");
static int m_nBody = utils->find_in_data_map(target->get_pred_desc_map(), "m_nBody");
info->render_info.origin = target->GetAbsOrigin();
info->render_info.angles = target->GetAbsAngles();
info->state.m_pStudioHdr = hdr;
info->state.m_pStudioHWData = interfaces->mdl_cache->GetHardwareData(model->studio);
info->state.m_pRenderable = renderable;
info->state.m_drawFlags = 0;
info->render_info.pRenderable = renderable;
info->render_info.pModel = model;
info->render_info.pLightingOffset = nullptr;
info->render_info.pLightingOrigin = nullptr;
info->render_info.hitboxset = target->get_hitbox_set();
info->render_info.skin = (int)(uintptr_t(target) + m_nSkin);
info->render_info.body = (int)(uintptr_t(target) + m_nBody);
info->render_info.entity_index = target->EntIndex();
info->render_info.instance = getvfunc<ModelInstanceHandle_t(__thiscall*)(void*) >(renderable, 30)(renderable);
info->render_info.flags = 0x1;
info->state.m_decals = 0;
info->state.m_lod = 0;
info->render_info.pModelToWorld = &info->cur_bones;
info->state.m_pModelToWorld = &info->cur_bones;
math::angle_matrix(info->render_info.angles, info->render_info.origin, info->cur_bones);
}
Код:
void draw_chams_on_shot() {
auto ctx = interfaces->material_system->GetRenderContext();
if (!ctx)
return;
for (int x = 0; x < 65; x++) {
auto& i = visuals->skeleton_info[x];
for (int s = 0; s < i.size(); s++) {
auto skelet = i[s];
float expire_time = interfaces->global_vars->curtime - skelet->time;
if (expire_time > 2.f)
skelet->alpha -= 1.f;
skelet->alpha = std::clamp(skelet->alpha, 0.f, 255.f);
if (skelet->alpha <= 0)
i.erase(i.begin() + s);
chams->material[2]->SetMaterialVarFlag(MATERIAL_VAR_IGNOREZ, true);
auto var3 = chams->material[2]->FindVar("$envmaptint", nullptr);
var3->set_vec_value(skelet->clr.r() / 255.f, skelet->clr.g() / 255.f, skelet->clr.b() / 255.f);
auto var2 = chams->material[2]->FindVar("$color", nullptr);
var2->set_vec_value(skelet->clr.r() / 255.f, skelet->clr.g() / 255.f, skelet->clr.b() / 255.f);
auto var5 = chams->material[2]->FindVar("$alpha", nullptr);
var5->set_float_value(skelet->alpha / 255.f);
interfaces->model_render->ForcedMaterialOverride(chams->material[2]);
interfaces->model_render->DrawModelExecute(ctx, skelet->state, skelet->render_info, skelet->bones);
interfaces->model_render->ForcedMaterialOverride(nullptr);
}
}
}
Код:
struct cache_user_t {
};
struct brushdata_t {
};
struct studiodata_t {
};
struct spritedata_t {
};
struct model_t
{
void* fnHandle;
char name[260];
__int32 nLoadFlags;
__int32 nServerCount;
__int32 type;
__int32 flags;
vec3 mins, maxs;
float radius;
void* m_pKeyValues;
union
{
brushdata_t brush;
MDLHandle_t studio;
spritedata_t sprite;
};
};
MODEL CACHE:
class IMdlCache : public c_app_system
{
public:
// Used to install callbacks for when data is loaded + unloaded
// Returns the prior notify
virtual void SetCacheNotify(void* pNotify) = 0;
// NOTE: This assumes the "GAME" path if you don't use
// the UNC method of specifying files. This will also increment
// the reference count of the MDL
virtual MDLHandle_t FindMDL(const char* pMDLRelativePath) = 0;
// Reference counting
virtual int AddRef(MDLHandle_t handle) = 0;
virtual int Release(MDLHandle_t handle) = 0;
virtual int GetRef(MDLHandle_t handle) = 0;
// Gets at the various data associated with a MDL
virtual studiohdr_t* GetStudioHdr(MDLHandle_t handle) = 0;
virtual studiohwdata_t* GetHardwareData(MDLHandle_t handle) = 0;
virtual vcollide_t* GetVCollide(MDLHandle_t handle) = 0;
virtual unsigned char* GetAnimBlock(MDLHandle_t handle, int nBlock) = 0;
virtual virtualmodel_t* GetVirtualModel(MDLHandle_t handle) = 0;
virtual int GetAutoplayList(MDLHandle_t handle, unsigned short** pOut) = 0;
virtual vertexFileHeader_t* GetVertexData(MDLHandle_t handle) = 0;
// Brings all data associated with an MDL into memory
virtual void TouchAllData(MDLHandle_t handle) = 0;
// Gets/sets user data associated with the MDL
virtual void SetUserData(MDLHandle_t handle, void* pData) = 0;
virtual void* GetUserData(MDLHandle_t handle) = 0;
// Is this MDL using the error model?
virtual bool IsErrorModel(MDLHandle_t handle) = 0;
// Flushes the cache, force a full discard
virtual void Flush(MDLCacheFlush_t nFlushFlags = MDLCACHE_FLUSH_ALL) = 0;
// Flushes a particular model out of memory
virtual void Flush(MDLHandle_t handle, int nFlushFlags = MDLCACHE_FLUSH_ALL) = 0;
// Returns the name of the model (its relative path)
virtual const char* GetModelName(MDLHandle_t handle) = 0;
// faster access when you already have the studiohdr
virtual virtualmodel_t* GetVirtualModelFast(const studiohdr_t* pStudioHdr, MDLHandle_t handle) = 0;
// all cache entries that subsequently allocated or successfully checked
// are considered "locked" and will not be freed when additional memory is needed
virtual void BeginLock() = 0;
// reset all protected blocks to normal
virtual void EndLock() = 0;
// returns a pointer to a counter that is incremented every time the cache has been out of the locked state (EVIL)
virtual int* GetFrameUnlockCounterPtrOLD() = 0;
// Finish all pending async operations
virtual void FinishPendingLoads() = 0;
virtual vcollide_t* GetVCollideEx(MDLHandle_t handle, bool synchronousLoad = true) = 0;
virtual bool GetVCollideSize(MDLHandle_t handle, int* pVCollideSize) = 0;
virtual bool GetAsyncLoad(MDLCacheDataType_t type) = 0;
virtual bool SetAsyncLoad(MDLCacheDataType_t type, bool bAsync) = 0;
virtual void BeginMapLoad() = 0;
virtual void EndMapLoad() = 0;
virtual void MarkAsLoaded(MDLHandle_t handle) = 0;
virtual void InitPreloadData(bool rebuild) = 0;
virtual void ShutdownPreloadData() = 0;
virtual bool IsDataLoaded(MDLHandle_t handle, MDLCacheDataType_t type) = 0;
virtual int* GetFrameUnlockCounterPtr(MDLCacheDataType_t type) = 0;
virtual studiohdr_t* LockStudioHdr(MDLHandle_t handle) = 0;
virtual void UnlockStudioHdr(MDLHandle_t handle) = 0;
virtual bool PreloadModel(MDLHandle_t handle) = 0;
// Hammer uses this. If a model has an error loading in GetStudioHdr, then it is flagged
// as an error model and any further attempts to load it will just get the error model.
// That is, until you call this function. Then it will load the correct model.
virtual void ResetErrorModelStatus(MDLHandle_t handle) = 0;
virtual void MarkFrame() = 0;
};
if (!mdl_cache)
mdl_cache = get_interfaces<IMdlCache>("datacache.dll", "MDLCache004");
Код:
struct skeleton_info_t
{
ModelRenderInfo_t render_info;
DrawModelState_t state;
matrix bones[128];
matrix cur_bones;
color clr;
float time;
float alpha;
skeleton_info_t() {
memset(bones, 0, sizeof(bones));
clr = color::White;
time = 0.f;
alpha = 255.f;
}
};
std::vector< skeleton_info_t* > skeleton_info[65];
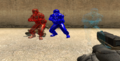
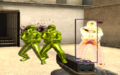