Тьомчик
-
Автор темы
- #1
C++:
void Visuals::BombTimer(C_BaseEntity* ent)
{
if (ent->GetClientClass()->m_ClassID == ClassId::ClassId_CPlantedC4) {
int x, y;
g_EngineClient->GetScreenSize(x, y);
float flblow = ent->m_flC4Blow();
float ExplodeTimeRemaining = flblow - (g_LocalPlayer->m_nTickBase() * g_GlobalVars->interval_per_tick);
float fldefuse = ent->m_flDefuseCountDown();
float DefuseTimeRemaining = fldefuse - (g_LocalPlayer->m_nTickBase() * g_GlobalVars->interval_per_tick);
char TimeToExplode[64]; sprintf_s(TimeToExplode, "Explode in: %.1f", ExplodeTimeRemaining);
char TimeToDefuse[64]; sprintf_s(TimeToDefuse, "Defuse in: %.1f", DefuseTimeRemaining);
if (ExplodeTimeRemaining > 0 && !ent->m_bBombDefused()) {
float fraction = ExplodeTimeRemaining / ent->m_flTimerLength();
int onscreenwidth = fraction * (x / 3);
// Background
g_VGuiSurface->DrawSetColor(0, 0, 0, 255);
g_VGuiSurface->DrawFilledRect(x / 3, y / 6, x / 3 * 2, y / 6 + 6);
// Filled Color
g_VGuiSurface->DrawSetColor(47, 154, 26, 255);
g_VGuiSurface->DrawFilledRect(x / 3, y / 6, x / 3 + onscreenwidth, y / 6 + 6);
// Outline
g_VGuiSurface->DrawSetColor(0, 0, 0, 255);
g_VGuiSurface->DrawOutlinedRect(x / 3, y / 6, x / 3 * 2, y / 6 + 6);
Render::Get().RenderText(TimeToExplode, ImVec2(x / 3 * 1.5, y / 6 - 12), 12, Color(255, 255, 255), true);
}
C_BasePlayer* Defuser = (C_BasePlayer*)C_BasePlayer::get_entity_from_handle(ent->m_hBombDefuser());
if (Defuser) {
float fraction = DefuseTimeRemaining / ent->m_flTimerLength();
int onscreenwidth = fraction * (x / 3);
// Background
g_VGuiSurface->DrawSetColor(30, 30, 30, 255);
g_VGuiSurface->DrawFilledRect(x / 3, y / 6 + 20, x / 3 * 2, y / 6 + 26);
// Filled Color
g_VGuiSurface->DrawSetColor(100, 255, 255, 255);
g_VGuiSurface->DrawFilledRect(x / 3, y / 6 + 20, x / 3 + onscreenwidth, y / 6 + 26);
// Outline
g_VGuiSurface->DrawSetColor(0, 0, 0, 255);
g_VGuiSurface->DrawOutlinedRect(x / 3, y / 6 + 20, x / 3 * 2, y / 6 + 26);
Render::Get().RenderText(TimeToDefuse, ImVec2(x / 3 * 1.5, y / 6 + 8), 12, Color(255, 255, 255), true);
}
}
}
C++:
class C_BaseEntity : public IClientEntity
{
public:
datamap_t * GetDataDescMap() {
typedef datamap_t*(__thiscall *o_GetPredDescMap)(void*);
return CallVFunction<o_GetPredDescMap>(this, 15)(this);
}
datamap_t *GetPredDescMap() {
typedef datamap_t*(__thiscall *o_GetPredDescMap)(void*);
return CallVFunction<o_GetPredDescMap>(this, 17)(this);
}
static __forceinline C_BaseEntity* GetEntityByIndex(int index) {
return static_cast<C_BaseEntity*>(g_EntityList->GetClientEntity(index));
}
static __forceinline C_BaseEntity* get_entity_from_handle(CBaseHandle h) {
return static_cast<C_BaseEntity*>(g_EntityList->GetClientEntityFromHandle(h));
}
NETVAR(int32_t, m_nModelIndex, "DT_BaseEntity", "m_nModelIndex");
NETVAR(int32_t, m_iTeamNum, "DT_BaseEntity", "m_iTeamNum");
NETVAR(Vector, m_vecOrigin, "DT_BaseEntity", "m_vecOrigin");
NETVAR(Vector, m_vecAngles, "DT_BaseEntity", "m_vecAngles");
NETVAR(bool, m_bShouldGlow, "DT_DynamicProp", "m_bShouldGlow");
NETVAR(CHandle<C_BasePlayer>, m_hOwnerEntity, "DT_BaseEntity", "m_hOwnerEntity");
NETVAR(bool, m_bSpotted, "DT_BaseEntity", "m_bSpotted");
NETVAR(float_t, m_flC4Blow, "DT_PlantedC4", "m_flC4Blow");
NETVAR(float_t, m_flDefuseCountDown, "DT_PlantedC4", "m_flDefuseCountDown");
NETVAR(bool, m_bBombDefused, "DT_PlantedC4", "m_bBombDefused");
NETVAR(CHandle<C_BasePlayer>, m_hBombDefuser, "DT_PlantedC4", "m_hBombDefuser");
NETVAR(float_t, m_flTimerLength, "DT_PlantedC4", "m_flTimerLength");
NETVAR(int, m_hRagdoll, "CCSPlayer", "m_hRagdoll")
const matrix3x4_t& m_rgflCoordinateFrame()
{
static auto _m_rgflCoordinateFrame = NetvarSys::Get().GetOffset("DT_BaseEntity", "m_CollisionGroup") - 0x30;
return *(matrix3x4_t*)((uintptr_t)this + _m_rgflCoordinateFrame);
}
bool IsPlayer();
bool IsLoot();
bool IsWeapon();
bool IsPlantedC4();
bool IsDefuseKit();
void setModelIndex(int modelIndex);
//bool isSpotted();
};
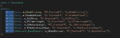