-
Автор темы
- #1
Обратите внимание, пользователь заблокирован на форуме. Не рекомендуется проводить сделки.
class
Код:
class C_Beam;
class Beam_t;
//---------------------------------------------------------------------------—
// Purpose: Popcorn trail for Beam Follow rendering...
//---------------------------------------------------------------------------—
enum
{
FBEAM_STARTENTITY = 0x00000001,
FBEAM_ENDENTITY = 0x00000002,
FBEAM_FADEIN = 0x00000004,
FBEAM_FADEOUT = 0x00000008,
FBEAM_SINENOISE = 0x00000010,
FBEAM_SOLID = 0x00000020,
FBEAM_SHADEIN = 0x00000040,
FBEAM_SHADEOUT = 0x00000080,
FBEAM_ONLYNOISEONCE = 0x00000100, // Only calculate our noise once
FBEAM_NOTILE = 0x00000200,
FBEAM_USE_HITBOXES = 0x00000400, // Attachment indices represent hitbox indices instead when this is set.
FBEAM_STARTVISIBLE = 0x00000800, // Has this client actually seen this beam's start entity yet?
FBEAM_ENDVISIBLE = 0x00001000, // Has this client actually seen this beam's end entity yet?
FBEAM_ISACTIVE = 0x00002000,
FBEAM_FOREVER = 0x00004000,
FBEAM_HALOBEAM = 0x00008000, // When drawing a beam with a halo, don't ignore the segments and endwidth
FBEAM_REVERSED = 0x00010000,
NUM_BEAM_FLAGS = 17 // KEEP THIS UPDATED!
};
enum
{
TE_BEAMPOINTS = 0x00, // beam effect between two points
TE_SPRITE = 0x01, // additive sprite, plays 1 cycle
TE_BEAMDISK = 0x02, // disk that expands to max radius over lifetime
TE_BEAMCYLINDER = 0x03, // cylinder that expands to max radius over lifetime
TE_BEAMFOLLOW = 0x04, // create a line of decaying beam segments until entity stops moving
TE_BEAMRING = 0x05, // connect a beam ring to two entities
TE_BEAMSPLINE = 0x06,
TE_BEAMRINGPOINT = 0x07,
TE_BEAMLASER = 0x08, // Fades according to viewpoint
TE_BEAMTESLA = 0x09,
};
struct BeamTrail_t
{
// NOTE: Don't add user defined fields except after these four fields.
BeamTrail_t* next;
float die;
Vector3 org;
Vector3 vel;
};
//---------------------------------------------------------------------------—
// Data type for beams.
//---------------------------------------------------------------------------—
struct BeamInfo_t
{
int m_nType;
// Entities
CPlayer* m_pStartEnt;
int m_nStartAttachment;
CPlayer* m_pEndEnt;
int m_nEndAttachment;
// Points
Vector3 m_vecStart;
Vector3 m_vecEnd;
int m_nModelIndex;
const char *m_pszModelName;
int m_nHaloIndex;
const char *m_pszHaloName;
float m_flHaloScale;
float m_flLife;
float m_flWidth;
float m_flEndWidth;
float m_flFadeLength;
float m_flAmplitude;
float m_flBrightness;
float m_flSpeed;
int m_nStartFrame;
float m_flFrameRate;
float m_flRed;
float m_flGreen;
float m_flBlue;
bool m_bRenderable;
int m_nSegments;
int m_nFlags;
// Rings
Vector3 m_vecCenter;
float m_flStartRadius;
float m_flEndRadius;
BeamInfo_t()
{
m_nType = TE_BEAMPOINTS;
m_nSegments = -1;
m_pszModelName = NULL;
m_pszHaloName = NULL;
m_nModelIndex = -1;
m_nHaloIndex = -1;
m_bRenderable = true;
m_nFlags = 0;
}
};
//---------------------------------------------------------------------------—
// Purpose: Declare client .dll beam entity interface
//---------------------------------------------------------------------------—
class IViewRenderBeams
{
public:
virtual void InitBeams(void) = 0;
virtual void ShutdownBeams(void) = 0;
virtual void ClearBeams(void) = 0;
// Updates the state of the temp ent beams
virtual void UpdateTempEntBeams() = 0;
virtual void DrawBeam(C_Beam* pbeam, ITraceFilter *pEntityBeamTraceFilter = NULL) = 0;
virtual void DrawBeam(Beam_t *pbeam) = 0;
virtual void KillDeadBeams(CPlayer *pEnt) = 0;
// New interfaces!
virtual Beam_t *CreateBeamEnts(BeamInfo_t &beamInfo) = 0;
virtual Beam_t *CreateBeamEntPoint(BeamInfo_t &beamInfo) = 0;
virtual Beam_t *CreateBeamPoints(BeamInfo_t &beamInfo) = 0;
virtual Beam_t *CreateBeamRing(BeamInfo_t &beamInfo) = 0;
virtual Beam_t *CreateBeamRingPoint(BeamInfo_t &beamInfo) = 0;
virtual Beam_t *CreateBeamCirclePoints(BeamInfo_t &beamInfo) = 0; [/CENTER]
[CENTER]virtual Beam_t *CreateBeamFollow(BeamInfo_t &beamInfo) = 0;
virtual void FreeBeam(Beam_t *pBeam) = 0;
virtual void UpdateBeamInfo(Beam_t *pBeam, BeamInfo_t &beamInfo) = 0;
// These will go away!
virtual void CreateBeamEnts(int startEnt, int endEnt, int modelIndex, int haloIndex, float haloScale,
float life, float width, float m_nEndWidth, float m_nFadeLength, float amplitude,
float brightness, float speed, int startFrame,
float framerate, float r, float g, float b, int type = -1) = 0;
virtual void CreateBeamEntPoint(int nStartEntity, const Vector3 *pStart, int nEndEntity, const Vector3* pEnd,
int modelIndex, int haloIndex, float haloScale,
float life, float width, float m_nEndWidth, float m_nFadeLength, float amplitude,
float brightness, float speed, int startFrame,
float framerate, float r, float g, float b) = 0;
virtual void CreateBeamPoints(Vector3& start, Vector3& end, int modelIndex, int haloIndex, float haloScale,
float life, float width, float m_nEndWidth, float m_nFadeLength, float amplitude,
float brightness, float speed, int startFrame,
float framerate, float r, float g, float b) = 0;
virtual void CreateBeamRing(int startEnt, int endEnt, int modelIndex, int haloIndex, float haloScale,
float life, float width, float m_nEndWidth, float m_nFadeLength, float amplitude,
float brightness, float speed, int startFrame,
float framerate, float r, float g, float b, int flags = 0) = 0;
virtual void CreateBeamRingPoint(const Vector3& center, float start_radius, float end_radius, int modelIndex, int haloIndex, float haloScale,
float life, float width, float m_nEndWidth, float m_nFadeLength, float amplitude,
float brightness, float speed, int startFrame,
float framerate, float r, float g, float b, int flags = 0) = 0;
virtual void CreateBeamCirclePoints(int type, Vector3& start, Vector3& end,
int modelIndex, int haloIndex, float haloScale, float life, float width,
float m_nEndWidth, float m_nFadeLength, float amplitude, float brightness, float speed,
int startFrame, float framerate, float r, float g, float b) = 0;
virtual void CreateBeamFollow(int startEnt, int modelIndex, int haloIndex, float haloScale,
float life, float width, float m_nEndWidth, float m_nFadeLength, float r, float g, float b,
float brightness) = 0;
};
Код:
IViewRenderBeams * ClientBeams;
интерфейс
extern IViewRenderBeams * ClientBeams;
Globals.ClientBeams = *reinterpret_cast<IViewRenderBeams**>(Globals.Misc->FindSignature("client.dll", "B9 ? ? ? ? A1 ? ? ? ? FF 10 A1 ? ? ? ? B9") + 1);
Код:
player_hurt_listener* hurtListener = new player_hurt_listener();
Interfaces.GameEventManager->AddListener(hurtListener, "bullet_impact", false);
Interfaces.GameEventManager->AddListener(hurtListener, "player_hurt", false);
Interfaces.GameEventManager->AddListener(hurtListener, "player_death", false);
сами поймете не тупие)
Код:
class CMyBulletTracer
{
public:
void CollectTracers(IGameEvent* pEvent);
void DrawTracers();
private:
class CMyBulletTracer_info
{
public:
CMyBulletTracer_info(Vector3 src, Vector3 dst, float time, Color colorLine ,Color ColorBox)
{
this->src = src;
this->dst = dst;
this->time = time;
this->color1 = colorLine;
this->color2 = ColorBox;
}
Vector3 src, dst;
float time;
Color color1;
Color color2;
};
std::vector<CMyBulletTracer_info> BulletTracerData;
};
CMyBulletTracer* BltTrc = new CMyBulletTracer();
enum CSWeaponType
{
WEAPONTYPE_KNIFE = 0,
WEAPONTYPE_PISTOL,
WEAPONTYPE_SUBMACHINEGUN,
WEAPONTYPE_RIFLE,
WEAPONTYPE_SHOTGUN,
WEAPONTYPE_SNIPER_RIFLE,
WEAPONTYPE_MACHINEGUN,
WEAPONTYPE_C4,
WEAPONTYPE_PLACEHOLDER,
WEAPONTYPE_GRENADE,
WEAPONTYPE_UNKNOWN
};
void CMyBulletTracer::CollectTracers(IGameEvent* pEvent)
{
//if we receive bullet_impact event
if (strstr(pEvent->GetName(), "bullet_impact"))
{
//get the user who fired the bullet
auto index = Interfaces.Engine->GetPlayerForUserID(pEvent->GetInt("userid"));
//return if the userid is not valid or we werent the entity who was fireing
if (index != Interfaces.Engine->GetLocalPlayer() && !Settings.Visuals.bAlwaysShowTracer)
return;
//get local player
CPlayer* pLocal;
bool bullshit = false;
if (Settings.Visuals.bAlwaysShowTracer)
{
pLocal = Interfaces.EntList->GetClientEntity<CPlayer>(index);
if (index != Interfaces.Engine->GetLocalPlayer())
bullshit = true;
}
else
{
pLocal = Interfaces.EntList->GetClientEntity<CPlayer>(Interfaces.Engine->GetLocalPlayer());
}
if (!pLocal)
return;
//get the bullet impact's position
Vector3 position(pEvent->GetInt("x"), pEvent->GetInt("y"), pEvent->GetInt("z"));
Ray_t ray;
ray.Init(pLocal->GetBestEyePos(bullshit), position);
//skip local player
CTraceFilter filter;
filter.pSkip = pLocal;
//trace a ray
trace_t tr;
Interfaces.EngineTrace->TraceRay(ray, MASK_SHOT, &filter, &tr);
//use different color when we hit a player
// Hit // NonHit
Color colorLine = (tr.m_pEnt && tr.hitgroup > 0 && tr.hitgroup <= 7) ? Color(Settings.Colors.BulletTracerLineHit.r, Settings.Colors.BulletTracerLineHit.g, Settings.Colors.BulletTracerLineHit.b) : Color(Settings.Colors.BulletTracerLineNonHit.r, Settings.Colors.BulletTracerLineNonHit.g, Settings.Colors.BulletTracerLineNonHit.b);
Color colorBox = (tr.m_pEnt && tr.hitgroup > 0 && tr.hitgroup <= 7) ? Color(Settings.Colors.BulletTracerBoxHit.r, Settings.Colors.BulletTracerBoxHit.g, Settings.Colors.BulletTracerBoxHit.b) : Color(Settings.Colors.BulletTracerBoxNonHit.r, Settings.Colors.BulletTracerBoxNonHit.g, Settings.Colors.BulletTracerBoxNonHit.b);
//push info to our vector
BulletTracerData.push_back(CMyBulletTracer_info(pLocal->GetBestEyePos(bullshit), position, Interfaces.Globals->curtime, colorLine, colorBox));
}
}
void CMyBulletTracer::DrawTracers()
{
if (Settings.Visuals.bBulletTracer) {
if (Interfaces.Engine->IsInGame() && Interfaces.Engine->IsConnected())
{
//get local player
CPlayer* pLocal = Interfaces.EntList->GetClientEntity<CPlayer>(Interfaces.Engine->GetLocalPlayer());
if (!pLocal)
return;
//get actual server time
float server_time = Hack.Misc->GetServerTime();
//loop through our vector
for (size_t i = 0; i < BulletTracerData.size(); i++)
{
//get the current item
auto current = BulletTracerData.at(i);
if (Settings.Visuals.bBeamBuletTracer) {
BeamInfo_t beamInfo;
beamInfo.m_nType = TE_BEAMPOINTS;
beamInfo.m_pszModelName = "sprites/physbeam.vmt";
beamInfo.m_nModelIndex = -1;
beamInfo.m_flHaloScale = 0.0f;
beamInfo.m_flLife = 1.f;
beamInfo.m_flWidth = 2.0f;
beamInfo.m_flEndWidth = 2.0f;
beamInfo.m_flFadeLength = 0.0f;
beamInfo.m_flAmplitude = 2.0f;
beamInfo.m_flBrightness = 255.f;
beamInfo.m_flSpeed = 0.2f;
beamInfo.m_nStartFrame = 0;
beamInfo.m_flFrameRate = 0.f;
beamInfo.m_flRed = current.color1.r();
beamInfo.m_flGreen = current.color1.g();
beamInfo.m_flBlue = current.color1.b();
beamInfo.m_nSegments = 2;
beamInfo.m_bRenderable = true;
beamInfo.m_nFlags = 0;
beamInfo.m_vecStart = current.src;
beamInfo.m_vecEnd = current.dst;
Beam_t* myBeam = Interfaces.ClientBeams->CreateBeamPoints(beamInfo);
if (myBeam)
{
Interfaces.ClientBeams->DrawBeam(myBeam);
}
}
else
{
//draw a line from local player's head position to the hit point
Interfaces.DebugOverlay->AddLineOverlay(current.src, current.dst, current.color1.r(), current.color1.g(), current.color1.b(), true, -1.0f);
//draw a box at the hit point
Interfaces.DebugOverlay->AddBoxOverlay(current.dst, Vector3(-2, -2, -2), Vector3(2, 2, 2), Vector3(0, 0, 0), current.color2.r(), current.color2.g(), current.color2.b() , 255, -1.0f);
}
//if the item is older than 5 seconds, delete it
if (fabs(server_time - current.time) > Settings.Visuals.flBulletTracerTime)
BulletTracerData.erase(BulletTracerData.begin() + i);
}
}
}
}
Код:
Vector3 CPlayer::GetBestEyePos(bool bUseGetBonePos)
{
if (Interfaces.Engine->IsInGame() && Interfaces.Engine->IsConnected()) {
if (this)
{
if (bUseGetBonePos)
{
return this->GetBonePosition(8);
}
else
{
return this->GetEyePosition();
}
}
}
return Vector3(0, 0, 0);
}
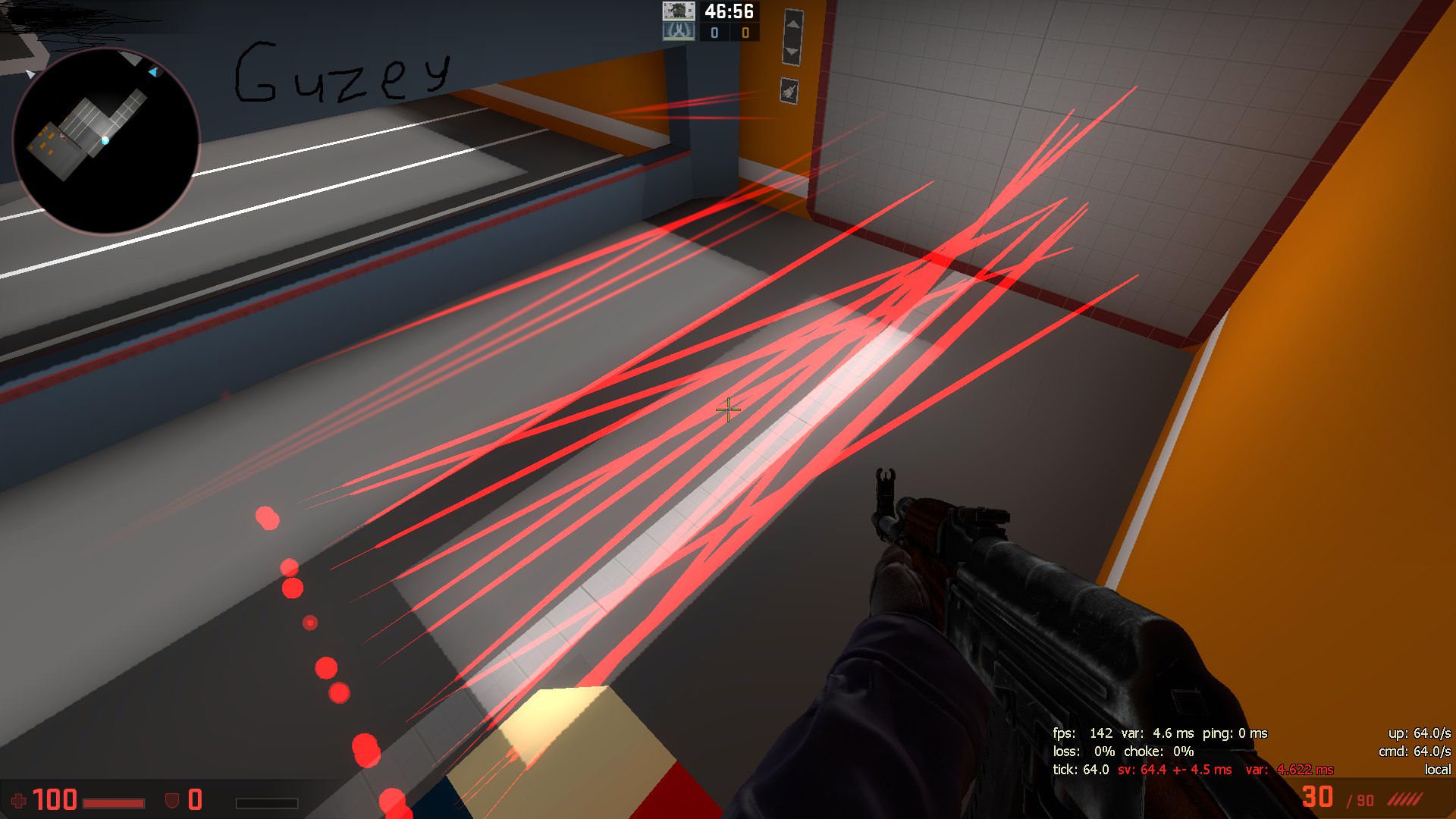
Последнее редактирование модератором: