-
Автор темы
- #1
C#:
private void OnGUI();
{
GUI.color = Color.white;
Circle(new Color32(255, 0, 0, 255), new Vector2(Screen.width / 2, Screen.height / 2), 100f );
}
public static void Circle(Color Col, Vector2 Center, float Radius)
{
GL.PushMatrix();
DrawMaterial.SetPass(0);
GL.Begin(1);
GL.Color(Col);
for (float num = 0f; num < 6.28318548f; num += 0.05f)
{
DrawLine(new Vector2(Mathf.Cos(num + 0.05f) * Radius + Center.x, Mathf.Sin(num + 0.05f) * Radius + Center.y), new Vector2(Mathf.Cos(num) * Radius + Center.x, Mathf.Sin(num) * Radius + Center.y),Color.red,1.3f,true);
}
GL.End();
GL.PopMatrix();
}
public static void DrawLine(Vector2 pointA, Vector2 pointB, Color color, float width, bool antiAlias)
{
float dx = pointB.x - pointA.x;
float dy = pointB.y - pointA.y;
float len = Mathf.Sqrt(dx * dx + dy * dy);
if (len < 0.001f)
{
return;
}
Texture2D tex;
Material mat;
if (antiAlias)
{
width = width * 3.0f;
tex = aaLineTex;
mat = blendMaterial;
}
else
{
tex = lineTex;
mat = blitMaterial;
}
float wdx = width * dy / len;
float wdy = width * dx / len;
Matrix4x4 matrix = Matrix4x4.identity;
matrix.m00 = dx;
matrix.m01 = -wdx;
matrix.m03 = pointA.x + 0.5f * wdx;
matrix.m10 = dy;
matrix.m11 = wdy;
matrix.m13 = pointA.y - 0.5f * wdy;
GL.PushMatrix();
GL.MultMatrix(matrix);
GUI.color = color;
GUI.DrawTexture(lineRect, tex);
GL.PopMatrix();
}
private static Rect lineRect = new Rect(0, 0, 1, 1);
public static Material DrawMaterial = new Material(Shader.Find("Hidden/Internal-Colored"));
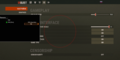
Последнее редактирование: