-
Автор темы
- #1
Шапка!
[DT]
[Cl_move]
[Can_fire]
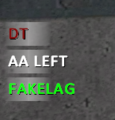
[DT]
C++:
bool misc::rapid_fire(CUserCmd* m_pcmd)
{
rapid_fire_enabled = true;
static auto recharge_rapid_fire = false;
static bool firing_dt = false;
static int last_double_tap = 0;
bool remove_knife = false;
if (recharge_rapid_fire)
{
recharge_rapid_fire = false;
recharging_rapid_fire = true;
globals.g.ticks_allowed = 0;
globals.g.next_tickbase_shift = 0;
return false;
}
auto max_tickbase_shift = globals.g.weapon->get_max_tickbase_shift();
if (recharging_rapid_fire)
{
bool in_attack = m_pcmd->m_buttons & IN_ATTACK;
bool can_recharge = globals.g.weapon->is_sniper() ? globals.g.weapon->can_fire(true, max_tickbase_shift)
: fabs(globals.g.fixed_tickbase - last_double_tap) > TIME_TO_TICKS(0.75f);
if (!in_attack && !aim::get().should_stop && can_recharge)
{
recharging_rapid_fire = false;
rapid_fire_key = true;
firing_dt = false;
}
else if (in_attack)
{
firing_dt = true;
last_double_tap = globals.g.fixed_tickbase;
}
}
if (!g_cfg.ragebot.enable)
{
rapid_fire_enabled = false;
rapid_fire_key = false;
globals.g.ticks_allowed = 0;
globals.g.next_tickbase_shift = 0;
return false;
}
if (!g_cfg.ragebot.double_tap)
{
rapid_fire_enabled = false;
rapid_fire_key = false;
globals.g.ticks_allowed = 0;
globals.g.next_tickbase_shift = 0;
return false;
}
static bool was_in_dt = false;
if (g_cfg.ragebot.double_tap_key.key <= KEY_NONE || g_cfg.ragebot.double_tap_key.key >= KEY_MAX)
{
rapid_fire_enabled = false;
rapid_fire_key = false;
globals.g.ticks_allowed = 0;
globals.g.next_tickbase_shift = 0;
return false;
}
if (rapid_fire_key && g_cfg.ragebot.double_tap_key.key != g_cfg.antiaim.hide_shots_key.key)
hide_shots_key = false;
if (!rapid_fire_key
|| globals.local()->m_bGunGameImmunity()
|| globals.local()->m_fFlags() & FL_FROZEN
|| globals.g.fakeducking)
{
rapid_fire_enabled = false;
if (!firing_dt && was_in_dt)
{
globals.g.trigger_teleport = true;
globals.g.teleport_amount = 14;
was_in_dt = false;
}
globals.g.ticks_allowed = 0;
globals.g.next_tickbase_shift = 0;
return false;
}
if (m_gamerules()->m_bIsValveDS())
{
rapid_fire_enabled = false;
globals.g.ticks_allowed = 0;
globals.g.next_tickbase_shift = 0;
return false;
}
if (globals.g.weapon->is_knife())
remove_knife = true;
if (antiaim::get().freeze_check)
return true;
was_in_dt = true;
bool can_peek = !globals.g.weapon->is_non_aim() && key_binds::get().get_key_bind_state(18);
if (!globals.g.weapon->is_grenade() && globals.g.weapon->m_iItemDefinitionIndex() != WEAPON_TASER
&& globals.g.weapon->m_iItemDefinitionIndex() != WEAPON_REVOLVER
&& (m_pcmd->m_buttons & IN_ATTACK || m_pcmd->m_buttons & IN_ATTACK2 && globals.g.weapon->is_knife())) //-V648
{
auto next_command_number = m_pcmd->m_command_number + 1;
auto user_cmd = m_input()->GetUserCmd(next_command_number);
memcpy(user_cmd, m_pcmd, sizeof(CUserCmd));
user_cmd->m_command_number = next_command_number;
util::copy_command(user_cmd, max_tickbase_shift);
globals.send_packet = true;
if (globals.g.aimbot_working)
{
globals.g.double_tap_aim = true;
globals.g.double_tap_aim_check = true;
}
recharge_rapid_fire = true;
rapid_fire_enabled = false;
rapid_fire_key = false;
last_double_tap = globals.g.fixed_tickbase;
firing_dt = true;
}
else if (!globals.g.weapon->is_grenade() && globals.g.weapon->m_iItemDefinitionIndex() != WEAPON_TASER && globals.g.weapon->m_iItemDefinitionIndex() != WEAPON_REVOLVER)
globals.g.tickbase_shift = max_tickbase_shift;
return true;
}
Код:
void __vectorcall hooks::hooked_clmove(float accumulated_extra_samples, bool bFinalTick)
{
((CLMove_t)original_clmove)(accumulated_extra_samples, bFinalTick);
if (globals.available() && globals.local()->is_alive())
{
if (globals.g.trigger_teleport)
{
for (int i = 0; i < globals.g.teleport_amount; i++)
((CLMove_t)original_clmove)(accumulated_extra_samples, bFinalTick);
globals.g.teleport_amount = 0;
globals.g.trigger_teleport = false;
}
}
}
Код:
bool weapon_t::can_fire(bool check_revolver, int tick_base)
{
if (!this) //-V704
return false;
if (!is_non_aim() && is_empty())
return false;
auto owner = (player_t*)m_entitylist()->GetClientEntityFromHandle(m_hOwnerEntity());
if (owner == globals.local() && antiaim::get().freeze_check)
return false;
if (!owner->valid(false))
return false;
if (owner->m_bIsDefusing())
return false;
auto server_time = TICKS_TO_TIME(globals.g.fixed_tickbase - tick_base);
if (server_time < m_flNextPrimaryAttack())
return false;
if (server_time < owner->m_flNextAttack())
return false;
if (check_revolver && m_iItemDefinitionIndex() == WEAPON_REVOLVER && m_flPostponeFireReadyTime() >= server_time)
return false;
return true;
}
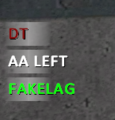
Последнее редактирование: