-
Автор темы
- #1
Перед прочтением основного контента ниже, пожалуйста, обратите внимание на обновление внутри секции Майна на нашем форуме. У нас появились:
- бесплатные читы для Майнкрафт — любое использование на свой страх и риск;
- маркетплейс Майнкрафт — абсолютно любая коммерция, связанная с игрой, за исключением продажи читов (аккаунты, предоставления услуг, поиск кодеров читов и так далее);
- приватные читы для Minecraft — в этом разделе только платные хаки для игры, покупайте группу "Продавец" и выставляйте на продажу свой софт;
- обсуждения и гайды — всё тот же раздел с вопросами, но теперь модернизированный: поиск нужных хаков, пати с игроками-читерами и другая полезная информация.
Спасибо!
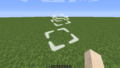
джампсерклы плывут, помогите решить плиз
код JumpCircles:
package fun.rich.feature.impl.visuals;
import com.mojang.blaze3d.matrix.MatrixStack;
import com.mojang.blaze3d.platform.GlStateManager;
import com.mojang.blaze3d.systems.RenderSystem;
import fun.rich.event.EventTarget;
import fun.rich.event.events.impl.player.PlayerJumpEvent;
import fun.rich.event.events.impl.player.WorldRenderEvent;
import fun.rich.event.events.impl.render.EventRender2D;
import fun.rich.event.events.impl.render.EventTick;
import fun.rich.feature.api.Module;
import fun.rich.feature.api.Category;
import fun.rich.feature.api.ModuleInfo;
import fun.rich.ui.settings.impl.NumberSetting;
import fun.rich.utils.animation.AnimationMath;
import fun.rich.utils.math.TimerUtil;
import fun.rich.utils.render.ColorUtils;
import lombok.Getter;
import lombok.Setter;
import net.minecraft.client.entity.player.ClientPlayerEntity;
import net.minecraft.client.renderer.ActiveRenderInfo;
import net.minecraft.client.renderer.BufferBuilder;
import net.minecraft.client.renderer.Tessellator;
import net.minecraft.util.ResourceLocation;
import net.minecraft.util.math.MathHelper;
import net.minecraft.util.math.vector.Quaternion;
import net.minecraft.util.math.vector.Vector3d;
import net.minecraft.util.math.vector.Vector3f;
import net.optifine.util.MathUtils;
import org.lwjgl.opengl.GL11;
import java.awt.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import static com.mojang.blaze3d.platform.GlStateManager.GL_QUADS;
import static com.mojang.blaze3d.systems.RenderSystem.*;
import static net.minecraft.client.renderer.vertex.DefaultVertexFormats.POSITION_TEX_COLOR;
@ModuleInfo(name = "Jump Circles", category = Category.Visuals)
[USER=270918]@Getter[/USER]
[USER=1132491]@setter[/USER]
public class JumpCircleModule extends Module {
private final List<Circle> circles = new ArrayList<>();
public JumpCircleModule() {
}
@Override
public void onDisable() {
super.onDisable();
circles.clear();
}
@EventTarget
public void onJump(PlayerJumpEvent evt) {
ClientPlayerEntity t = mc.player;
if (mc.player.isOnGround()) {
if (circles.size() > 20) circles.remove(0);
this.circles.add(new Circle(new Vector3d(t.getPosX(), t.getPosY(), t.getPosZ()), (long) (10000.0f), this.circles.size()));
}
}
@EventTarget
public void onPreTick(EventTick event) {
circles.removeIf(c -> c.timer.hasReached((int)c.time));
}
public static MatrixStack matrixFrom(MatrixStack matrices, ActiveRenderInfo camera) {
matrices.rotate(Vector3f.XP.rotationDegrees(camera.getPitch()));
matrices.rotate(Vector3f.YP.rotationDegrees(camera.getYaw() + 180.0F));
return matrices;
}
@EventTarget
public void onRender(WorldRenderEvent evt) {
//render preview
MatrixStack ms = matrixFrom(evt.getMatrixStack(),mc.gameRenderer.getActiveRenderInfo());
double ix = -((mc.getRenderManager().info.getProjectedView().getX()));
double iy = -((mc.getRenderManager().info.getProjectedView().getY()));
double iz = -((mc.getRenderManager().info.getProjectedView().getZ()));
Collections.reverse(circles);
//circles
for (Circle c : circles) {
double x = c.positionVector.x - c.factor / 2;
double y = c.positionVector.y;
double z = c.positionVector.z - c.factor / 2;
c.factor = fun.rich.utils.render.MathUtils.lerp(c.factor, 2, 0.15f);
c.alpha = fun.rich.utils.render.MathUtils.lerp(c.alpha, 0, 0.015f);
Tessellator tessellator = Tessellator.getInstance();
BufferBuilder bufferbuilder = tessellator.getBuffer();
ms.push();
ms.translate(ix, iy, iz);
ms.translate(x, y, z);
ms.rotate(new Quaternion(new Vector3f(1, 0, 0), 90, true));
enableBlend();
disableAlphaTest();
depthMask(false);
float rsp = (float) (c.timer.getTimePassed()) / (float) (c.time);
GL11.glAlphaFunc(GL11.GL_GREATER, 0.02f);
GL11.glDisable(GL11.GL_POINT_SMOOTH);
float apc = 1.0f - rsp;
apc = (double) apc >= 0.85 ? (1.0f - apc) * 6 : apc;
apc = (float) fun.rich.utils.render.MathUtils.easeInOutQuad(apc, 1);
apc = MathHelper.clamp(apc, 0.0f, 1.0f);
int a = (int) MathHelper.clamp(apc * 255.0f, 0.0f, 255.0f);
Color colorI = Hud.getColor(1);
Color colorB = Hud.getColor(50);
colorI = Color.RED;
colorB = Hud.getColor(1);
int bs = 2;
int bs2 = 0;
bs = bs * 4;
float b = 0.5f;
int finalBs = bs;
boolean renderColor = true;
if (renderColor) {
colorB = Color.WHITE;
colorI = Color.WHITE;
}
int color = ColorUtils.withAlpha(colorB, (int) (a)).getRGB();
int color2 = ColorUtils.withAlpha(colorI, a).getRGB();
enableBlend();
RenderSystem.blendFuncSeparate(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA, GL11.GL_ONE, GL11.GL_ZERO);
RenderSystem.enableDepthTest();
//render
//file position
mc.getTextureManager().bindTexture(new ResourceLocation("rich/icons/target.png"));
bufferbuilder.begin(GL_QUADS, POSITION_TEX_COLOR);
bufferbuilder.pos(ms.getLast().getMatrix(), 0, 0, 0).tex(0, 0).color(
ColorUtils.r(color), ColorUtils.g(color), ColorUtils.b(color), ColorUtils.a(color)
).endVertex();
bufferbuilder.pos(ms.getLast().getMatrix(),0, c.factor, 0).tex(0, 1).color(
ColorUtils.r(color2), ColorUtils.g(color2), ColorUtils.b(color2), ColorUtils.a(color)).endVertex();
bufferbuilder.pos(ms.getLast().getMatrix(),c.factor, c.factor, 0).tex(1, 1).color(
ColorUtils.r(color), ColorUtils.g(color), ColorUtils.b(color), ColorUtils.a(color)).endVertex();
bufferbuilder.pos(ms.getLast().getMatrix(),c.factor, 0, 0).tex(1, 0).color(
ColorUtils.r(color), ColorUtils.g(color), ColorUtils.b(color), ColorUtils.a(color)).endVertex();
tessellator.draw();
enableAlphaTest();
disableBlend();
depthMask(true);
ms.pop();
}
Collections.reverse(circles);
};
//xui ego znaet
public class Circle {
public Vector3d positionVector;
public long time;
public TimerUtil timer;
public int index;
public float alpha = 255, factor = 0;
public boolean reached = false;
public Circle(Vector3d pos, long time, int index) {
this.positionVector = pos;
this.time = time;
this.index = index;
this.timer = new TimerUtil();
}
}
}