-
Автор темы
- #1
Хз сливали или нет, самопис
Итог:
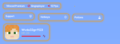
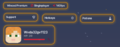
DisplayUtils:
private static final Tessellator tessellator = Tessellator.getInstance();
private static final BufferBuilder buffer = tessellator.getBuffer();
public static float[] getColorComponents(Color color) {
return new float[] {
color.getRed() / 255f,
color.getGreen() / 255f,
color.getBlue() / 255f,
color.getAlpha() / 255f
};
}
public static void drawGradientOutlineRoundedRect(float x, float y, float width, float height, Vector4f roundingVec, float lineWidth, int... colors) {
GlStateManager.pushMatrix();
ShaderUtil.setupRenderState();
ShaderUtil.roundedout.attach();
ShaderUtil.roundedout.setUniformf("size", width, height);
ShaderUtil.roundedout.setUniformf("round", roundingVec.getX(), roundingVec.getY(), roundingVec.getZ(), roundingVec.getW());
ShaderUtil.roundedout.setUniformf("lineWidth", lineWidth);
ShaderUtil.roundedout.setUniformf("smoothness", 0.0f, 1.5f);
float[] color1 = ColorUtils.rgba(colors[0]);
float[] color2 = ColorUtils.rgba(colors[1]);
float[] color3 = ColorUtils.rgba(colors[2]);
float[] color4 = ColorUtils.rgba(colors[3]);
ShaderUtil.roundedout.setUniformf("colorOutline1", color1[0], color1[1], color1[2], color1[3]);
ShaderUtil.roundedout.setUniformf("colorOutline2", color2[0], color2[1], color2[2], color2[3]);
ShaderUtil.roundedout.setUniformf("colorOutline3", color3[0], color3[1], color3[2], color3[3]);
ShaderUtil.roundedout.setUniformf("colorOutline4", color4[0], color4[1], color4[2], color4[3]);
buffer.begin(GL11.GL_QUADS, DefaultVertexFormats.POSITION);
buffer.pos(x, y, 0).endVertex();
buffer.pos(x, y + height, 0).endVertex();
buffer.pos(x + width, y + height, 0).endVertex();
buffer.pos(x + width, y, 0).endVertex();
tessellator.draw();
ShaderUtil.roundedout.detach();
ShaderUtil.cleanup();
GlStateManager.popMatrix();
}
public static void drawRoundedOutline(double x, double y, double width, double height, double radius, float lineWidth, Color color) {
GlStateManager.pushMatrix();
ShaderUtil.setupRenderState();
glEnable(GL_LINE_SMOOTH);
glHint(GL_LINE_SMOOTH_HINT, GL_NICEST);
GL11.glLineWidth(lineWidth);
float[] c = getColorComponents(color);
GL11.glColor4f(c[0], c[1], c[2], c[3]);
double x2 = x + width;
double y2 = y + height;
buffer.begin(GL_LINE_LOOP, DefaultVertexFormats.POSITION);
int segments = 36;
double theta = Math.PI / 2 / segments;
for (int i = 0; i <= segments; i++) {
buffer.pos(x2 - radius + radius * Math.cos(theta * i), y + radius - radius * Math.sin(theta * i), 0).endVertex();
}
for (int i = 0; i <= segments; i++) {
buffer.pos(x + radius - radius * Math.sin(theta * i), y + radius - radius * Math.cos(theta * i), 0).endVertex();
}
for (int i = 0; i <= segments; i++) {
buffer.pos(x + radius - radius * Math.cos(theta * i), y2 - radius + radius * Math.sin(theta * i), 0).endVertex();
}
for (int i = 0; i <= segments; i++) {
buffer.pos(x2 - radius + radius * Math.sin(theta * i), y2 - radius + radius * Math.cos(theta * i), 0).endVertex();
}
tessellator.draw();
glDisable(GL_LINE_SMOOTH);
GL11.glLineWidth(1.0f);
ShaderUtil.cleanup();
GlStateManager.popMatrix();
}
public static void drawShadowOutline(double x, double y, double width, double height, double radius, float lineWidth, int shadowRadius, Color outlineColor) {
float shadowX = (float)x - shadowRadius;
float shadowY = (float)y - shadowRadius;
float shadowWidth = (float)width + shadowRadius * 2;
float shadowHeight = (float)height + shadowRadius * 2;
int identifier = Objects.hash(shadowWidth, shadowHeight, shadowRadius, lineWidth);
int textureId;
if (shadowCache.containsKey(identifier)) {
textureId = shadowCache.get(identifier);
GlStateManager.bindTexture(textureId);
} else {
BufferedImage originalImage = new BufferedImage((int) shadowWidth, (int) shadowHeight, BufferedImage.TYPE_INT_ARGB_PRE);
Graphics2D graphics = originalImage.createGraphics();
graphics.setColor(Color.WHITE);
graphics.setStroke(new BasicStroke(lineWidth));
graphics.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
graphics.drawRoundRect(shadowRadius, shadowRadius, (int)(width), (int)(height), (int)(radius * 2), (int)(radius * 2));
graphics.dispose();
GaussianFilter filter = new GaussianFilter(shadowRadius);
BufferedImage blurredImage = filter.filter(originalImage, null);
DynamicTexture texture = new DynamicTexture(TextureUtils.toNativeImage(blurredImage));
texture.setBlurMipmap(false, false);
textureId = texture.getGlTextureId();
shadowCache.put(identifier, textureId);
}
GlStateManager.enableBlend();
GlStateManager.blendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA);
GlStateManager.alphaFunc(GL_GREATER, 0.01f);
GlStateManager.disableAlphaTest();
float[] shadowColorComps = getColorComponents(outlineColor);
shadowColorComps[3] = 0.25f;
buffer.begin(GL_QUADS, DefaultVertexFormats.POSITION_COLOR_TEX);
buffer.pos(shadowX, shadowY, 0)
.color(shadowColorComps[0], shadowColorComps[1], shadowColorComps[2], shadowColorComps[3])
.tex(0, 0).endVertex();
buffer.pos(shadowX, shadowY + shadowHeight, 0)
.color(shadowColorComps[0], shadowColorComps[1], shadowColorComps[2], shadowColorComps[3])
.tex(0, 1).endVertex();
buffer.pos(shadowX + shadowWidth, shadowY + shadowHeight, 0)
.color(shadowColorComps[0], shadowColorComps[1], shadowColorComps[2], shadowColorComps[3])
.tex(1, 1).endVertex();
buffer.pos(shadowX + shadowWidth, shadowY, 0)
.color(shadowColorComps[0], shadowColorComps[1], shadowColorComps[2], shadowColorComps[3])
.tex(1, 0).endVertex();
tessellator.draw();
GlStateManager.enableAlphaTest();
GlStateManager.bindTexture(0);
GlStateManager.disableBlend();
}
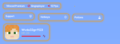
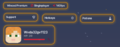
Последнее редактирование: