-
Автор темы
- #1
Даю общий доступ к моему коду для написания ESP.
Для справки, соответствующие идентификаторы костей соответствуют этим местам;
В сущности вы будете должны прочитать ComponentToWorld что FTransform внутри USkeletalMeshComponent.
Он не определен в SDK, поэтому вам придется найти его вручную.
ComponentToWorld = AActor + 0x400] + 0x190
Вот мой внешний метод GetBoneIndex.
Это будет читать отдельные вектора костей из массива, который содержит все кости.
Массив называется "Cachedbone Space Transforms", но SDK не имеет никаких ссылок на него, поэтому вам придется найти это вручную, если он изменился.
Это получает местоположение каждой из костей.
Последние идентификаторы костей:
Объедините их в скелет. (каждый массив является частью, которая должна быть нарисована индивидуально)
И, наконец, провести линии между каждой костью..
У вас будет что-то вроде этого
Для справки, соответствующие идентификаторы костей соответствуют этим местам;
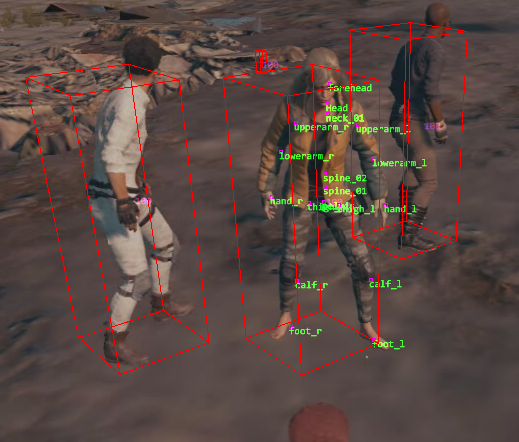
В сущности вы будете должны прочитать ComponentToWorld что FTransform внутри USkeletalMeshComponent.
Он не определен в SDK, поэтому вам придется найти его вручную.
ComponentToWorld = AActor + 0x400] + 0x190
Код:
actor.ComponentToWorld = ReadFTransform(mesh+ 0x190);
Код:
public class FTransform
{
public Vector4 Rotation;
public Vector3 Translation;
public Vector3 Scale3D;
public FTransform(Vector4 rot, Vector3 translation, Vector3 scale)
{
Rotation = rot;
Translation = translation;
Scale3D = scale;
}
public Matrix ToMatrixWithScale()
{
Matrix m = new Matrix();
m.M41 = Translation.X;
m.M42 = Translation.Y;
m.M43 = Translation.Z;
float x2 = Rotation.X + Rotation.X;
float y2 = Rotation.Y + Rotation.Y;
float z2 = Rotation.Z + Rotation.Z;
float xx2 = Rotation.X * x2;
float yy2 = Rotation.Y * y2;
float zz2 = Rotation.Z * z2;
m.M11 = (1.0f - (yy2 + zz2)) * Scale3D.X;
m.M22 = (1.0f - (xx2 + zz2)) * Scale3D.Y;
m.M33 = (1.0f - (xx2 + yy2)) * Scale3D.Z;
float yz2 = Rotation.Y * z2;
float wx2 = Rotation.W * x2;
m.M32 = (yz2 - wx2) * Scale3D.Z;
m.M23 = (yz2 + wx2) * Scale3D.Y;
float xy2 = Rotation.X * y2;
float wz2 = Rotation.W * z2;
m.M21 = (xy2 - wz2) * Scale3D.Y;
m.M12 = (xy2 + wz2) * Scale3D.X;
float xz2 = Rotation.X * z2;
float wy2 = Rotation.W * y2;
m.M31 = (xz2 + wy2) * Scale3D.Z;
m.M13 = (xz2 - wy2) * Scale3D.X;
m.M14 = 0.0f;
m.M24 = 0.0f;
m.M34 = 0.0f;
m.M44 = 1.0f;
return m;
}
}
Код:
public FTransform ReadFTransform(long ptr)
{
//i know this is shitty. I still dont care.
float quatX = ReadFloat(ptr + 0x00);
float quatY = ReadFloat(ptr + 0x04);
float quatZ = ReadFloat(ptr + 0x08);
float quatW = ReadFloat(ptr + 0x0C);
float transX = ReadFloat(ptr + 0x0010);
float transY = ReadFloat(ptr + 0x0014);
float transZ = ReadFloat(ptr + 0x0018);
float scaleX = ReadFloat(ptr + 0x0020);
float scaleY = ReadFloat(ptr + 0x0024);
float scaleZ = ReadFloat(ptr + 0x0028);
Vector4 fQuat = new Vector4(quatW, quatX, quatY, quatZ);
Vector3 trans = new Vector3(transX, transY, transZ);
Vector3 scale = new Vector3(scaleX, scaleY, scaleZ);
FTransform fTransform = new FTransform(fQuat, trans, scale);
return fTransform;
}
Это будет читать отдельные вектора костей из массива, который содержит все кости.
Массив называется "Cachedbone Space Transforms", но SDK не имеет никаких ссылок на него, поэтому вам придется найти это вручную, если он изменился.
Код:
public FTransform GetBoneIndex(long actorPtr, int boneIndex)
{
long mesh = ReadInt64(actorPtr + 0x400); //USkeletalMeshComponent
long arrayBones = ReadInt64(mesh + 0x0790); //CachedBoneSpaceTransforms
var transform = ReadFTransform(arrayBones + (boneIndex * 0x30));
return transform;
}
Код:
public Vector3 GetBoneWithRotation(int id, ArrayActorInfo actor)
{
var bone = GetBoneIndex(actor.BasePointer , id); //get the bone vector without any rotation applied
var boneMatrix = bone.ToMatrixWithScale();
var componentToWorldMatrix = actor.ComponentToWorld.ToMatrixWithScale(); //bone translations are applied to ComponentToWorld matrix
var newMatrix = boneMatrix * componentToWorldMatrix;
return new Vector3(newMatrix.M41, newMatrix.M42, newMatrix.M43);
}
Код:
enum Bone : int
{
Root = 0,
pelvis = 1,
spine_01 = 2,
spine_02 = 3,
spine_03 = 4,
neck_01 = 5,
Head = 6,
face_root = 7,
eyebrows_pos_root = 8,
eyebrows_root = 9,
eyebrows_r = 10,
eyebrows_l = 11,
eyebrow_l = 12,
eyebrow_r = 13,
forehead_root = 14,
forehead = 15,
jaw_pos_root = 16,
jaw_root = 17,
jaw = 18,
mouth_down_pos_root = 19,
mouth_down_root = 20,
lip_bm_01 = 21,
lip_bm_02 = 22,
lip_br = 23,
lip_bl = 24,
jaw_01 = 25,
jaw_02 = 26,
cheek_pos_root = 27,
cheek_root = 28,
cheek_r = 29,
cheek_l = 30,
nose_side_root = 31,
nose_side_r_01 = 32,
nose_side_r_02 = 33,
nose_side_l_01 = 34,
nose_side_l_02 = 35,
eye_pos_r_root = 36,
eye_r_root = 37,
eye_rot_r_root = 38,
eye_lid_u_r = 39,
eye_r = 40,
eye_lid_b_r = 41,
eye_pos_l_root = 42,
eye_l_root = 43,
eye_rot_l_root = 44,
eye_lid_u_l = 45,
eye_l = 46,
eye_lid_b_l = 47,
nose_pos_root = 48,
nose = 49,
mouth_up_pos_root = 50,
mouth_up_root = 51,
lip_ul = 52,
lip_um_01 = 53,
lip_um_02 = 54,
lip_ur = 55,
lip_l = 56,
lip_r = 57,
hair_root = 58,
hair_b_01 = 59,
hair_b_02 = 60,
hair_l_01 = 61,
hair_l_02 = 62,
hair_r_01 = 63,
hair_r_02 = 64,
hair_f_02 = 65,
hair_f_01 = 66,
hair_b_pt_01 = 67,
hair_b_pt_02 = 68,
hair_b_pt_03 = 69,
hair_b_pt_04 = 70,
hair_b_pt_05 = 71,
camera_fpp = 72,
GunReferencePoint = 73,
GunRef = 74,
breast_l = 75,
breast_r = 76,
clavicle_l = 77,
upperarm_l = 78,
lowerarm_l = 79,
hand_l = 80,
thumb_01_l = 81,
thumb_02_l = 82,
thumb_03_l = 83,
thumb_04_l_MBONLY = 84,
index_01_l = 85,
index_02_l = 86,
index_03_l = 87,
index_04_l_MBONLY = 88,
middle_01_l = 89,
middle_02_l = 90,
middle_03_l = 91,
middle_04_l_MBONLY = 92,
ring_01_l = 93,
ring_02_l = 94,
ring_03_l = 95,
ring_04_l_MBONLY = 96,
pinky_01_l = 97,
pinky_02_l = 98,
pinky_03_l = 99,
pinky_04_l_MBONLY = 100,
item_l = 101,
lowerarm_twist_01_l = 102,
upperarm_twist_01_l = 103,
clavicle_r = 104,
upperarm_r = 105,
lowerarm_r = 106,
hand_r = 107,
thumb_01_r = 108,
thumb_02_r = 109,
thumb_03_r = 110,
thumb_04_r_MBONLY = 111,
index_01_r = 112,
index_02_r = 113,
index_03_r = 114,
index_04_r_MBONLY = 115,
middle_01_r = 116,
middle_02_r = 117,
middle_03_r = 118,
middle_04_r_MBONLY = 119,
ring_01_r = 120,
ring_02_r = 121,
ring_03_r = 122,
ring_04_r_MBONLY = 123,
pinky_01_r = 124,
pinky_02_r = 125,
pinky_03_r = 126,
pinky_04_r_MBONLY = 127,
item_r = 128,
lowerarm_twist_01_r = 129,
upperarm_twist_01_r = 130,
BackPack = 131,
backpack_01 = 132,
backpack_02 = 133,
Slot_Primary = 134,
Slot_Secondary = 135,
Slot_Melee = 136,
slot_throwable = 137,
coat_l_01 = 138,
coat_l_02 = 139,
coat_l_03 = 140,
coat_l_04 = 141,
coat_fl_01 = 142,
coat_fl_02 = 143,
coat_fl_03 = 144,
coat_fl_04 = 145,
coat_b_01 = 146,
coat_b_02 = 147,
coat_b_03 = 148,
coat_b_04 = 149,
coat_r_01 = 150,
coat_r_02 = 151,
coat_r_03 = 152,
coat_r_04 = 153,
coat_fr_01 = 154,
coat_fr_02 = 155,
coat_fr_03 = 156,
coat_fr_04 = 157,
thigh_l = 158,
calf_l = 159,
foot_l = 160,
ball_l = 161,
calf_twist_01_l = 162,
thigh_twist_01_l = 163,
thigh_r = 164,
calf_r = 165,
foot_r = 166,
ball_r = 167,
calf_twist_01_r = 168,
thigh_twist_01_r = 169,
Slot_SideArm = 170,
skirt_l_01 = 171,
skirt_l_02 = 172,
skirt_l_03 = 173,
skirt_f_01 = 174,
skirt_f_02 = 175,
skirt_f_03 = 176,
skirt_b_01 = 177,
skirt_b_02 = 178,
skirt_b_03 = 179,
skirt_r_01 = 180,
skirt_r_02 = 181,
skirt_r_03 = 182,
ik_hand_root = 183,
ik_hand_gun = 184,
ik_hand_r = 185,
ik_hand_l = 186,
ik_aim_root = 187,
ik_aim_l = 188,
ik_aim_r = 189,
ik_foot_root = 190,
ik_foot_l = 191,
ik_foot_r = 192,
camera_tpp = 193,
ik_target_root = 194,
ik_target_l = 195,
ik_target_r = 196,
VB_spine_03_spine_03 = 197,
VB_upperarm_r_lowerarm_r = 198
}
Код:
static Bone[ ] skeletonUpper = new Bone[ ] {
Bone.forehead, Bone.Head, Bone.neck_01,
};
static Bone[ ] skeletonRightArm = new Bone[ ] {
Bone.neck_01, Bone.upperarm_r, Bone.lowerarm_r, Bone.hand_r
};
static Bone[ ] skeletonLeftArm = new Bone[ ] {
Bone.neck_01, Bone.upperarm_l, Bone.lowerarm_l, Bone.hand_l
};
static Bone[ ] skeletonSpine = new Bone[ ] {
Bone.neck_01, Bone.spine_01, Bone.spine_02, Bone.pelvis
};
static Bone[ ] skeletonLowerRight = new Bone[ ] {
Bone.pelvis, Bone.thigh_r, Bone.calf_r, Bone.foot_r
};
static Bone[ ] skeletonLowerLeft = new Bone[ ] {
Bone.pelvis, Bone.thigh_l, Bone.calf_l, Bone.foot_l
};
static Bone[ ][ ] skeleton = new Bone[ ][ ] {
skeletonUpper, skeletonLeftArm, skeletonRightArm, skeletonSpine, skeletonLowerRight, skeletonLowerLeft
};
Код:
foreach (Bone[ ] part in skeleton)
{
Vector3 previousBone = new Vector3();
foreach (Bone b in part)
{
var currentBone = GetBoneWithRotation((int)b, actor);
if (previousBone.X == 0) //first bone no draw happens
{
previousBone = currentBone;
continue;
}
else
{
//draw line from previousBone to currentBone W2S
DrawLine3D(previousBone, currentBone);
previousBone = currentBone;
}
}
}
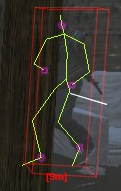