-
Автор темы
- #1
Обратите внимание, пользователь заблокирован на форуме. Не рекомендуется проводить сделки.
Hello, may I ask why the menu is coming pixel by pixel as I have shown in the photo, how can I solve it?
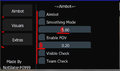
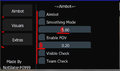
C++:
#pragma once
#include <Windows.h>
#include <string>
#include <iostream>
#include <Psapi.h>
#include <vector>
#include <map>
#include <fstream>
#include <tchar.h>
#include <sstream>
#include <GL/gl.h>
#include <GL/glu.h>
#include "MinHook/MinHook.h"
#include "ImGUI/imgui.h"
#include "ImGUI/imgui_impl_opengl2.h"
#include "ImGUI/imgui_impl_win32.h"
#include "Cheats/CheatIncludes.hpp"
#include "ImGUI/imgui_internal.h"
#pragma comment(lib, "opengl32.lib")
#pragma comment(lib, "glu32.lib")
#pragma comment(lib, "D3dcompiler.lib")
#pragma comment(lib, "d3d11.lib")
#define _WIN32_WINNT 0x0500
#define WINDOW_WIDTH 335
#define WINDOW_HEIGHT 450
bool aimbot = false;
bool FOVCircle = false;
float Sensitivity = 0.2;
int FOV = 120;
float SmoothValue = 5.0f;
bool UIIsCurrentlyOpen;
int esp{ 0 };
using namespace std;
namespace Variables {
int ActiveTab = 1;
}
std::hash<std::string> HashString;
HMODULE SorpModule;
void(*Orig_SwapBuffers)(HDC dc) = nullptr;
template <typename T>
inline MH_STATUS MH_CreateHookApiEx(LPCWSTR pszModule, LPCSTR pszProcName, LPVOID pDetour, T** ppOriginal)
{
return MH_CreateHookApi(pszModule, pszProcName, pDetour, reinterpret_cast<LPVOID*>(ppOriginal));
}
void OpenGLDraw(RECT Rect) {
DWORD window_flags = ImGuiWindowFlags_NoResize | ImGuiWindowFlags_NoSavedSettings | ImGuiWindowFlags_NoCollapse | ImGuiWindowFlags_NoScrollbar;
auto& IO = ImGui::GetIO();
IO.DisplaySize.x = Rect.right - Rect.left;
IO.DisplaySize.y = Rect.bottom - Rect.top;
IO.Fonts->AddFontFromFileTTF("C:\\Windows\\Fonts\\Tahoma.ttf", 16.0f);
ImGui_ImplWin32_NewFrame();
ImGui_ImplOpenGL2_NewFrame();
ImGui::NewFrame();
{
ImGui::SetNextWindowSize(ImVec2(400, 250));
ImGui::SetNextWindowBgAlpha(1.0f);
ImGui::Begin("Noise", 0, window_flags);
{
ImGui::Columns(2);
ImGui::SetColumnOffset(1, 120);
ImGui::Spacing();
if (ImGui::Button("Aimbot", ImVec2(100, 40)))
Variables::ActiveTab = 1;
ImGui::Spacing();
if (ImGui::Button("Visuals", ImVec2(100, 40)))
Variables::ActiveTab = 2;
ImGui::Spacing();
if (ImGui::Button("Extras", ImVec2(100, 40)))
Variables::ActiveTab = 3;
ImGui::Spacing();
ImGui::Spacing();
ImGui::Spacing();
ImGui::Spacing();
ImGui::Spacing();
ImGui::Spacing();
ImGui::Spacing();
ImGui::Spacing();
ImGui::Spacing();
ImGui::Spacing();
ImGui::Text(" Made By");
ImGui::Text("NotSlater#0999");
ImGui::NextColumn();
{
if (Variables::ActiveTab == 1)
{
ImGui::Text(" -=Aimbot=-");
ImGui::Spacing();
ImGui::Checkbox("Aimbot", &aimbot);
ImGui::Checkbox("Smoothing Mode", &aimbot);
ImGui::SliderFloat(" ", &SmoothValue, 1, 10, "% .2f");
ImGui::Checkbox("Enable FOV", &FOVCircle);
ImGui::SliderFloat("", &Sensitivity, 0, 240, "% .2f");
ImGui::Checkbox("Visible Check", &aimbot);
ImGui::Checkbox("Team Check", &aimbot);
}
if (Variables::ActiveTab == 2)
{
ImGui::Text(" -=Visuals=-");
ImGui::Spacing();
ImGui::Checkbox("ESP", &aimbot);
ImGui::Checkbox("Full Box", &aimbot);
ImGui::Checkbox("Corner Box", &aimbot);
ImGui::Checkbox("Skeleton", &aimbot);
ImGui::Checkbox("Player Name", &aimbot);
ImGui::Checkbox("Player Health", &aimbot);
ImGui::Checkbox("Player Weapon", &aimbot);
}
if (Variables::ActiveTab == 3)
{
ImGui::Text(" -=Extras=-");
ImGui::Checkbox("No Recoil", &aimbot);
ImGui::SliderFloat(" ", &Sensitivity, 0, 100, "% .2f");
ImGui::Checkbox("NoNoSpreadSpread", &aimbot);
ImGui::SliderFloat(" ", &Sensitivity, 0, 100, "% .2f");
}
}
}
ImGui::End();
}
ImGui::EndFrame();
ImGui::Render();
glClear(256);
glMatrixMode(5889);
glOrtho(0.0f, (int)IO.DisplaySize.x, (int)IO.DisplaySize.y, 0.0f, 0.0f, 100.0f);
glViewport(0, 0, (int)IO.DisplaySize.x, (int)IO.DisplaySize.y);
ImGui_ImplOpenGL2_RenderDrawData(ImGui::GetDrawData());
}
static void SwapOpenGLBuffers(HDC DC) {
if (!DC) {
return Orig_SwapBuffers(DC);
}
if (UIIsCurrentlyOpen) {
HWND const WND = WindowFromDC(DC);
RECT WNDRect;
GetClientRect(WND, &WNDRect);
OpenGLDraw(WNDRect);
}
return Orig_SwapBuffers(DC);
}
typedef LRESULT(CALLBACK*OWindowProc) (
_In_ HWND hwnd,
_In_ UINT uMsg,
_In_ WPARAM wParam,
_In_ LPARAM lParam
);
extern LRESULT ImGui_ImplWin32_WndProcHandler(HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam);
OWindowProc WNDProc = nullptr;
bool TabBackAutoClick;
static LRESULT WINAPI HWNDProcHandle(HWND hWnd, UINT Msg, WPARAM wParam, LPARAM lParam) {
DWORD Proc;
auto& IO = ImGui::GetIO();
if (UIIsCurrentlyOpen) {
if (ImGui_ImplWin32_WndProcHandler(hWnd, Msg, wParam, lParam)) {
return TRUE;
}
}
switch (Msg) {
case WM_KEYUP: {
if (wParam == VK_SHIFT)
{
UIIsCurrentlyOpen =! UIIsCurrentlyOpen;
if (UIIsCurrentlyOpen) {
TabBackAutoClick = true;
ReleaseCapture();
}
if (!UIIsCurrentlyOpen) {
if (TabBackAutoClick) {
//use this to auto click back into game upon UI closing so you can look around again without having to click
INPUT Input = { 0 };
Input.type = INPUT_MOUSE;
Input.mi.dwFlags = MOUSEEVENTF_LEFTDOWN;
SendInput(1, &Input, sizeof(INPUT));
ZeroMemory(&Input, sizeof(INPUT));
Input.type = INPUT_MOUSE;
Input.mi.dwFlags = MOUSEEVENTF_LEFTUP;
SendInput(1, &Input, sizeof(INPUT));
TabBackAutoClick = false;
}
}
}
}
case WM_SETCURSOR:
{
if (UIIsCurrentlyOpen) {
return 0;
}
}
}
ret:
return CallWindowProc(WNDProc, hWnd, Msg, wParam, lParam);
}
LRESULT CALLBACK DXGIMsgProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam) { return DefWindowProc(hwnd, uMsg, wParam, lParam); }
void InitGUI() {
ImGui::CreateContext();
auto MineCraftWindow = FindWindow(_T("LWJGL"), NULL);
ImGui_ImplWin32_Init(MineCraftWindow);
ImGui_ImplOpenGL2_Init();
WNDProc = (OWindowProc)SetWindowLongPtr(MineCraftWindow, GWLP_WNDPROC, (LONG_PTR)HWNDProcHandle);
ImGuiStyle& style = ImGui::GetStyle();
ImGui::GetStyle().FrameRounding = 4.0f;
ImGui::GetStyle().GrabRounding = 4.0f;
ImVec4* colors = ImGui::GetStyle().Colors;
colors[ImGuiCol_Text] = ImVec4(0.95f, 0.96f, 0.98f, 1.00f);
colors[ImGuiCol_TextDisabled] = ImVec4(0.36f, 0.42f, 0.47f, 1.00f);
colors[ImGuiCol_WindowBg] = ImColor(10, 10, 10, 255);
colors[ImGuiCol_ChildBg] = ImVec4(0.15f, 0.18f, 0.22f, 1.00f);
colors[ImGuiCol_PopupBg] = ImVec4(0.08f, 0.08f, 0.08f, 0.94f);
colors[ImGuiCol_Border] = ImVec4(0.08f, 0.10f, 0.12f, 1.00f);
colors[ImGuiCol_BorderShadow] = ImVec4(0.00f, 0.00f, 0.00f, 0.00f);
colors[ImGuiCol_FrameBg] = ImVec4(0.20f, 0.25f, 0.29f, 1.00f);
colors[ImGuiCol_FrameBgHovered] = ImVec4(0.12f, 0.20f, 0.28f, 1.00f);
colors[ImGuiCol_FrameBgActive] = ImVec4(0.09f, 0.12f, 0.14f, 1.00f);
colors[ImGuiCol_TitleBg] = ImColor(30, 30, 160, 255);
colors[ImGuiCol_TitleBgActive] = ImColor(30, 30, 160, 255);
colors[ImGuiCol_TitleBgCollapsed] = ImColor(0, 0, 0, 130);
colors[ImGuiCol_MenuBarBg] = ImVec4(0.15f, 0.18f, 0.22f, 1.00f);
colors[ImGuiCol_ScrollbarBg] = ImColor(31, 30, 31, 255);
colors[ImGuiCol_ScrollbarGrab] = ImColor(41, 40, 41, 255);
colors[ImGuiCol_ScrollbarGrabHovered] = ImColor(31, 30, 31, 255);
colors[ImGuiCol_ScrollbarGrabActive] = ImColor(41, 40, 41, 255);
colors[ImGuiCol_CheckMark] = ImColor(10, 10, 200, 255);
colors[ImGuiCol_SliderGrab] = ImColor(180, 30, 31, 255);
colors[ImGuiCol_SliderGrabActive] = ImColor(185, 35, 35, 255);
colors[ImGuiCol_Button] = ImColor(31, 30, 31, 255);
colors[ImGuiCol_ButtonHovered] = ImColor(31, 30, 31, 255);
colors[ImGuiCol_ButtonActive] = ImColor(41, 40, 41, 255);
colors[ImGuiCol_Header] = ImVec4(0.20f, 0.25f, 0.29f, 0.55f);
colors[ImGuiCol_HeaderHovered] = ImVec4(0.26f, 0.59f, 0.98f, 0.80f);
colors[ImGuiCol_HeaderActive] = ImVec4(0.26f, 0.59f, 0.98f, 1.00f);
colors[ImGuiCol_Separator] = ImVec4(0.20f, 0.25f, 0.29f, 1.00f);
colors[ImGuiCol_SeparatorHovered] = ImVec4(0.10f, 0.40f, 0.75f, 0.78f);
colors[ImGuiCol_SeparatorActive] = ImVec4(0.10f, 0.40f, 0.75f, 1.00f);
colors[ImGuiCol_ResizeGrip] = ImVec4(0.26f, 0.59f, 0.98f, 0.25f);
colors[ImGuiCol_ResizeGripHovered] = ImVec4(0.26f, 0.59f, 0.98f, 0.67f);
colors[ImGuiCol_ResizeGripActive] = ImVec4(0.26f, 0.59f, 0.98f, 0.95f);
colors[ImGuiCol_Tab] = ImVec4(0.11f, 0.15f, 0.17f, 1.00f);
colors[ImGuiCol_TabHovered] = ImVec4(0.26f, 0.59f, 0.98f, 0.80f);
colors[ImGuiCol_TabActive] = ImVec4(0.20f, 0.25f, 0.29f, 1.00f);
colors[ImGuiCol_TabUnfocused] = ImVec4(0.11f, 0.15f, 0.17f, 1.00f);
colors[ImGuiCol_TabUnfocusedActive] = ImVec4(0.11f, 0.15f, 0.17f, 1.00f);
colors[ImGuiCol_PlotLines] = ImVec4(0.61f, 0.61f, 0.61f, 1.00f);
colors[ImGuiCol_PlotLinesHovered] = ImVec4(1.00f, 0.43f, 0.35f, 1.00f);
colors[ImGuiCol_PlotHistogram] = ImVec4(0.90f, 0.70f, 0.00f, 1.00f);
colors[ImGuiCol_PlotHistogramHovered] = ImVec4(1.00f, 0.60f, 0.00f, 1.00f);
colors[ImGuiCol_TextSelectedBg] = ImVec4(0.26f, 0.59f, 0.98f, 0.35f);
colors[ImGuiCol_DragDropTarget] = ImVec4(1.00f, 1.00f, 0.00f, 0.90f);
colors[ImGuiCol_NavHighlight] = ImVec4(0.26f, 0.59f, 0.98f, 1.00f);
colors[ImGuiCol_NavWindowingHighlight] = ImVec4(1.00f, 1.00f, 1.00f, 0.70f);
colors[ImGuiCol_NavWindowingDimBg] = ImVec4(0.80f, 0.80f, 0.80f, 0.20f);
colors[ImGuiCol_ModalWindowDimBg] = ImVec4(0.80f, 0.80f, 0.80f, 0.35f);
if (MH_Initialize() != MH_OK) {
MessageBox(NULL, _T("Unable To Initiate BloxxHaxx UI! Error Code: 1"), _T("Fatal Error!"), MB_OK | MB_ICONERROR);
exit(0);
}
if (MH_CreateHookApiEx(L"Gdi32.dll", "SwapBuffers", &SwapOpenGLBuffers, &Orig_SwapBuffers) != MH_OK) {
MessageBox(NULL, _T("Unable To Initiate BloxxHaxx UI! Error Code: 2"), _T("Fatal Error!"), MB_OK | MB_ICONERROR);
exit(0);
}
if (MH_EnableHook(MH_ALL_HOOKS)) {
MessageBox(NULL, _T("Unable To Initiate BloxxHaxx UI! Error Code: 3"), _T("Fatal Error!"), MB_OK | MB_ICONERROR);
exit(0);
}
}